React JS Beginner to Advanced series (2)
Understanding JSX, Components, and Props
What is JSX?
JSX stands for JavaScript XML. It's a syntax extension for JavaScript, often used with React to describe what the UI should look like. It looks like HTML but ultimately gets transformed into regular JavaScript function calls.
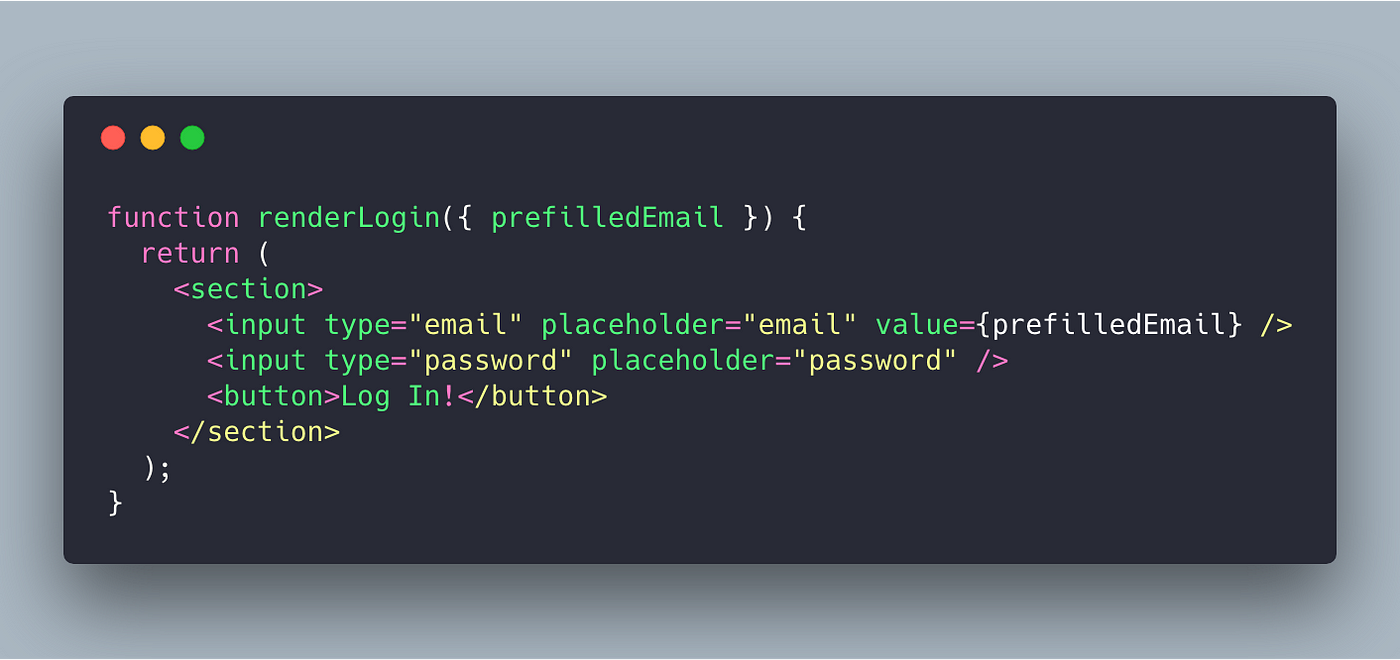
Understanding Components
In React, components are the building blocks of UI. They are reusable and encapsulate the logic and UI into small, manageable pieces. There are two types of components: functional components and class components.
Component Lifecycle
Components go through a series of lifecycle phases from initialization to unmounting. Understanding the component lifecycle is essential for managing state, performing side effects, and optimizing performance.e.
Initialization
- constructor: The constructor is called before a component is mounted. It is used for initializing state and binding event handlers.
- getDerivedStateFromProps: This static method is invoked right before calling the
render
method, both on the initial mount and on subsequent updates. It should return an object to update the state, or null to indicate that the new props do not require any state updates.
Mounting
- componentWillMount (deprecated): Executed just before rendering occurs on both the client and server-side.
- render: The
render
method is required and is responsible for returning the JSX that represents the component. - componentDidMount: This method is called after the component is mounted to the DOM. It is used for performing any side effects such as fetching data from APIs or setting up subscriptions.
Updating
- componentWillReceiveProps (deprecated): Invoked before a mounted component receives new props. Not called for the initial render.
- shouldComponentUpdate: Determines if the component should re-render after receiving new props or state. Used for performance optimization.
- componentWillUpdate (deprecated): Called just before rendering when new props or state are being received.
- render: The
render
method is called to update the component UI. - componentDidUpdate: Invoked immediately after updating occurs. Used for performing side effects such as DOM manipulations or network requests.
Unmounting
- componentWillUnmount: Called just before the component is unmounted and destroyed. Used for cleanup tasks such as removing event listeners or canceling subscriptions.
Types of Components
Components in React can be broadly classified into two types: functional components and class components. Functional components are JavaScript functions that take props as input and return React elements. Class components are ES6 classes that extend React.Component and have a render method.

Props
In React, props (short for properties) are a way to pass data from parent components to child components. Props are read-only and cannot be modified by the child components.
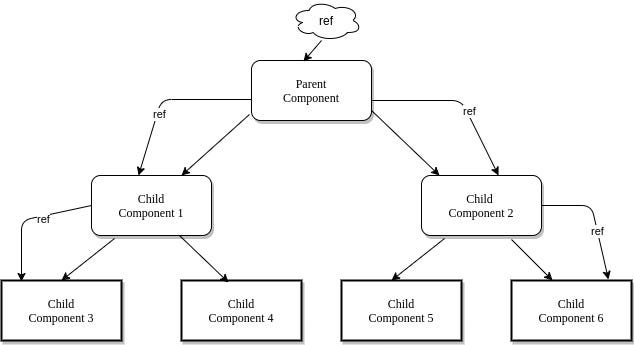
Understanding Props:
- Parent Component Renders: The parent component renders and includes the child component.
- Parent Passes Props: The parent component passes props to the child component by specifying them as attributes in the child component tag.
- Child Receives Props: The child component receives the props passed by the parent component through its props parameter.
- Child Uses Props: The child component can access and use the props received from the parent component to render its content.
More to Go
Thank you for reading my second blog post in the ReactJS Beginner to Advanced series! In the next post, we'll deep dive into more advanced topics like handling events, updating component state, and conditional rendering. Stay tuned for more insightful content!